java解析xml特殊字符的方法
java解析xml特殊字符的方法

推荐答案
在Java中,解析XML字符串时需要特别处理包含特殊字符的情况。XML特殊字符包括<、>、&、'和"。当这些字符出现在XML中时,需要进行转义以确保XML的正确性。
以下是一种常用的处理方法,使用javax.xml.transform.TransformerFactory和javax.xml.transform.Transformer来进行转义和反转义XML特殊字符:
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.w3c.dom.Document;
public class XmlSpecialCharacterExample {
public static void main(String[] args) throws Exception {
String xmlString = "Foo & Bar";
// 将XML字符串解析为Document对象
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(new InputSource(new StringReader(xmlString)));
// 创建Transformer对象,并指定特性进行转义
TransformerFactory transformerFactory = TransformerFactory.newInstance();
Transformer transformer = transformerFactory.newTransformer();
transformer.setOutputProperty(OutputKeys.ENCODING, "UTF-8");
transformer.setOutputProperty(OutputKeys.INDENT, "yes");
transformer.setOutputProperty(OutputKeys.OMIT_XML_DECLARATION, "yes");
// 创建DOMSource和StreamResult对象
DOMSource source = new DOMSource(document);
StreamResult result = new StreamResult(System.out);
// 转义XML特殊字符
transformer.transform(source, result);
}
}
上述代码将输出以下内容:
Foo & Bar
在以上代码中,我们首先使用DocumentBuilder将XML字符串解析为Document对象,然后创建一个Transformer对象,指定输出特性。最后,我们使用transform()方法将DOMSource对象转化为StreamResult对象,并输出转义后的XML。
通过这种方式,我们可以确保XML字符串中的特殊字符正确转义,从而保持XML解析的正确性。

热议问题
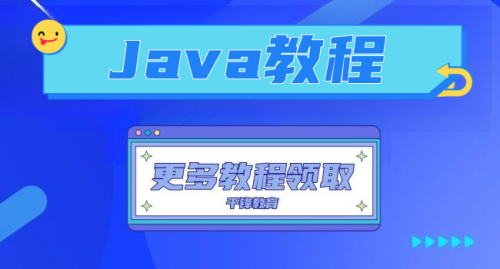
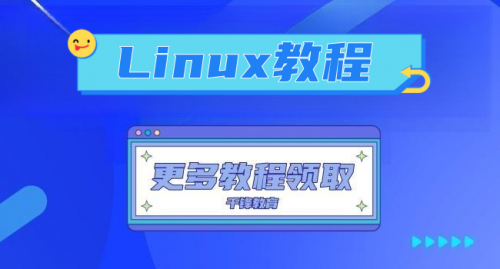
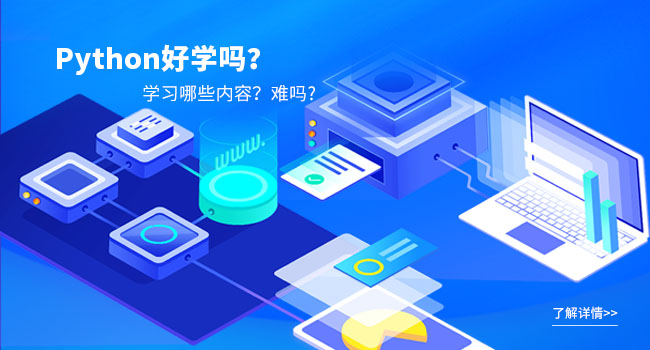
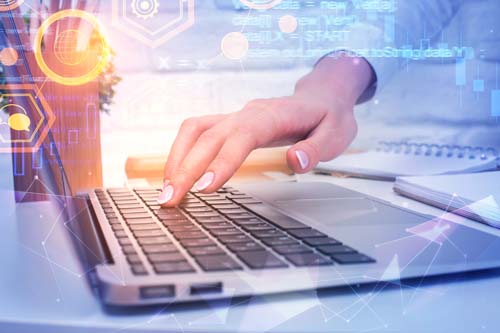
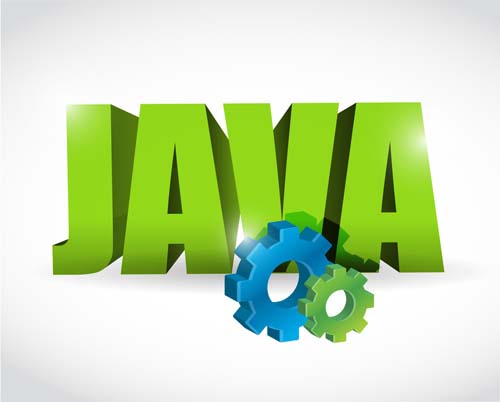
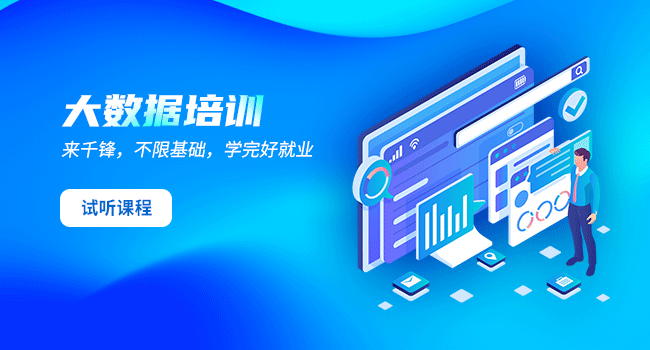
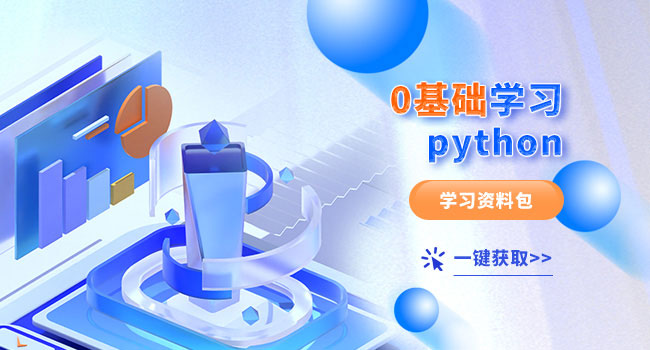